faldornMar Abr 30, 2013 9:34 am
Nuevo Usuario
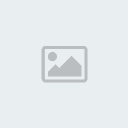
En rpg maker xp hay un script que hace lo mismo que el caterpillar pero en lugar de poner los "followers" en linea recta los pone dispersos, en formación de escuadrón. Aquí os pongo una imagen:

Y aquí el código:

Y aquí el código:
- Spoiler:
#======================================
# ■ Squad Movement
# By: Near Fantastica
# Date: 23.05.05
#
# Controls
# Q - Change Party Lead Forward
# W - change Party Lead Backwards
# A - Player Wait
# S - Gather Actors
#======================================
#======================================
# ■ Game_Party
#======================================
class Game_Party
#--------------------------------------------------------------------------
alias abs_game_party_initialize initialize
#--------------------------------------------------------------------------
attr_accessor :party_max
#--------------------------------------------------------------------------
def initialize
@party_max = 6
@player_trans = false
abs_game_party_initialize
end
#--------------------------------------------------------------------------
def add_actor(actor_id)
actor = $game_actors[actor_id]
if @actors.size < @party_max and not @actors.include?(actor)
@actors.push(actor)
$game_player.refresh
id = @actors.size-1
$game_allies[id] = Game_Ally.new(id)
$game_allies[id].refresh
$game_allies[id].moveto($game_player.x,$game_player.y)
if $scene.spriteset != nil
$scene.spriteset.add_ally(id)
end
end
end
#--------------------------------------------------------------------------
def remove_actor(actor_id)
actor = $game_actors[actor_id]
if @actors.include?(actor)
@actors.delete(actor)
$game_player.refresh
id = $game_allies.length
temp_ally = $game_allies[id]
$game_allies.delete(id)
if $scene.spriteset != nil
$scene.spriteset.remove_ally
end
for ally in $game_allies.values
ally.refresh
end
end
end
#--------------------------------------------------------------------------
def shift(direction)
if direction == "Left"
shift_forward
elsif direction == "Right"
shift_backward
end
end
#--------------------------------------------------------------------------
def shift_forward
temp = @actors.shift
@actors.push(temp)
$game_player.refresh
for ally in $game_allies.values
ally.refresh
end
if $game_party.actors.size <= 1
return
end
x = $game_player.x
y = $game_player.y
direction = $game_player.direction
wait = $game_player.wait_command
map_id = $game_player.map_id
transparent = $game_player.transparent
$game_player.moveto($game_allies[1].x,$game_allies[1].y)
$game_player.direction = $game_allies[1].direction
$game_player.wait_command = false
$game_player.map_id = $game_allies[1].map_id
$game_player.transparent = false
@player_trans = $game_allies[1].transparent
for i in 1...$game_party.actors.size-1
$game_allies[i].moveto($game_allies[i+1].x,$game_allies[i+1].y)
$game_allies[i].direction = $game_allies[i+1].direction
$game_allies[i].wait_command = $game_allies[i+1].wait_command
$game_allies[i].map_id = $game_allies[i+1].map_id
$game_allies[i].transparent = $game_allies[i+1].transparent
end
$game_allies[$game_party.actors.size-1].moveto(x,y)
$game_allies[$game_party.actors.size-1].direction = direction
$game_allies[$game_party.actors.size-1].wait_command = wait
$game_allies[$game_party.actors.size-1].map_id = map_id
$game_allies[$game_party.actors.size-1].transparent = @player_trans
if $game_player.map_id != $game_map.map_id
$game_map.setup($game_player.map_id)
$game_map.autoplay
$game_map.update
for ally in $game_allies.values
if ally.map_id != $game_map.map_id
ally.wait_command = true
end
end
$scene = Scene_Map.new
end
end
#--------------------------------------------------------------------------
def shift_backward
temp = @actors.pop
@actors.unshift(temp)
$game_player.refresh
for ally in $game_allies.values
ally.refresh
end
if $game_party.actors.size <= 1
return
end
x = $game_player.x
y = $game_player.y
direction = $game_player.direction
wait = $game_player.wait_command
map_id = $game_player.map_id
transparent = $game_player.transparent
id = $game_party.actors.size-1
$game_player.moveto($game_allies[id].x, $game_allies[id].y)
$game_player.direction = $game_allies[id].direction
$game_player.wait_command = false
$game_player.map_id = $game_allies[id].map_id
$game_player.transparent = false
@player_trans = $game_allies[id].transparent
for i in 1...$game_party.actors.size-1
id = $game_party.actors.size-i
$game_allies[id].moveto($game_allies[id -1].x,$game_allies[id-1].y)
$game_allies[id].direction = $game_allies[id -1].direction
$game_allies[id].wait_command = $game_allies[id -1].wait_command
$game_allies[id].map_id = $game_allies[id -1].map_id
$game_allies[id].transparent = $game_allies[id -1].transparent
end
$game_allies[1].moveto(x,y)
$game_allies[1].direction = direction
$game_allies[1].wait_command = wait
$game_allies[1].map_id = map_id
$game_allies[1].transparent = @player_trans
if $game_player.map_id != $game_map.map_id
$game_map.setup($game_player.map_id)
$game_map.autoplay
$game_map.update
for ally in $game_allies.values
if ally.map_id != $game_map.map_id
ally.wait_command = true
else
ally.wait_command = false
end
end
$scene = Scene_Map.new
end
end
end
#==============================================================================
# ■ Game_Character
#==============================================================================
class Game_Character
#--------------------------------------------------------------------------
attr_accessor :character_name
attr_accessor :character_hue
attr_accessor :direction
attr_accessor :wait_command
attr_accessor :map_id
#--------------------------------------------------------------------------
alias abs_game_character_initialize initialize
#--------------------------------------------------------------------------
def initialize
abs_game_character_initialize
@wait_command = false
@map_id = 0
end
#--------------------------------------------------------------------------
def move_ally(ally)
ally.move_away_from_player
end
#--------------------------------------------------------------------------
def passable?(x, y, d)
new_x = x + (d == 6 ? 1 : d == 4 ? -1 : 0)
new_y = y + (d == 2 ? 1 : d == 8 ? -1 : 0)
unless $game_map.valid?(new_x, new_y)
return false
end
if @through
return true
end
unless $game_map.passable?(x, y, d, self)
return false
end
unless $game_map.passable?(new_x, new_y, 10 - d)
return false
end
for event in $game_map.events.values
if event.x == new_x and event.y == new_y
unless event.through
if self != $game_player
return false
end
if event.character_name != ""
return false
end
end
end
end
for ally in $game_allies.values
if ally.map_id == $game_map.map_id
if ally.x == new_x and ally.y == new_y
unless ally.through
if self != $game_player
return false
else
move_ally(ally)
return true
end
if ally.character_name != ""
return false
end
end
end
end
end
if $game_player.x == new_x and $game_player.y == new_y
unless $game_player.through
if @character_name != ""
return false
end
end
end
return true
end
end
#=====================================
# ■ Game_Ally
#=====================================
class Game_Ally < Game_Character
#--------------------------------------------------------------------------
attr_accessor :actor_id
#--------------------------------------------------------------------------
def initialize(actor_id)
super()
@actor_id = actor_id
@map_id = $game_player.map_id
refresh
end
#--------------------------------------------------------------------------
def refresh
if $game_party.actors.size == 0
@character_name = ""
@character_hue = 0
return
end
if $game_party.actors[@actor_id] == nil
@character_name = ""
@character_hue = 0
return
end
actor = $game_party.actors[@actor_id]
@character_name = actor.character_name
@character_hue = actor.character_hue
@opacity = 255
@blend_type = 0
end
#--------------------------------------------------------------------------
def update
if @wait_command == false and @map_id == $game_map.map_id
if !moving?
if $game_party.actors[@actor_id] == nil
return
end
case $data_classes[$game_party.actors[@actor_id].class_id].position
when 0
if !in_range?(2)
move_toward_player
end
when 1
if !in_range?(3)
move_toward_player
end
when 2
if !in_range?(4)
move_toward_player
end
end
end
super
end
end
#--------------------------------------------------------------------------
def in_range?(range)
playerx = $game_player.x
playery = $game_player.y
x = (playerx - @x) * (playerx - @x)
y = (playery - @y) * (playery - @y)
r = x +y
if r <= (range * range)
return true
else
return false
end
end
#--------------------------------------------------------------------------
def facing_player?
playerx = $game_player.x
playery = $game_player.y
if event_direction == 2
if playery >= @y
return true
end
end
if event_direction == 4
if playerx <= @x
return true
end
end
if event_direction == 6
if playerx >= @x
return true
end
end
if event_direction == 8
if playery <= @y
return true
end
end
return false
end
#--------------------------------------------------------------------------
def check_event_trigger_touch(x, y)
return false
end
end
#=====================================
# ■ Game_Map
#=====================================
class Game_Map
#--------------------------------------------------------------------------
def passable?(x, y, d, self_event = nil)
unless valid?(x, y)
return false
end
bit = (1 << (d / 2 - 1)) & 0x0f
for event in events.values
if event.tile_id >= 0 and event != self_event and
event.x == x and event.y == y and not event.through
if @passages[event.tile_id] & bit != 0
return false
elsif @passages[event.tile_id] & 0x0f == 0x0f
return false
elsif @priorities[event.tile_id] == 0
return true
end
end
end
for ally in $game_allies.values
if ally.map_id == $game_map.map_id
if ally.tile_id >= 0 and ally != self_event and
ally.x == x and ally.y == y and not ally.through
if @passages[ally.tile_id] & bit != 0
return false
elsif @passages[ally.tile_id] & 0x0f == 0x0f
return false
elsif @priorities[ally.tile_id] == 0
return true
end
end
end
end
for i in [2, 1, 0]
tile_id = data[x, y, i]
if tile_id == nil
return false
elsif @passages[tile_id] & bit != 0
return false
elsif @passages[tile_id] & 0x0f == 0x0f
return false
elsif @priorities[tile_id] == 0
return true
end
end
return true
end
end
#======================================
# ■ Spriteset_Map
#======================================
class Spriteset_Map
#--------------------------------------------------------------------------
alias abs_spriteset_map_initialize initialize
#--------------------------------------------------------------------------
def initialize
abs_spriteset_map_initialize
for ally in $game_allies.values
if ally.map_id != $game_map.map_id
ally.transparent = true
else
ally.transparent = false
end
sprite = Sprite_Character.new(@viewport1, ally)
@character_sprites.push(sprite)
end
end
#--------------------------------------------------------------------------
def add_ally(id)
sprite = Sprite_Character.new(@viewport1, $game_allies[id])
@character_sprites.push(sprite)
end
#--------------------------------------------------------------------------
def remove_ally
index = @character_sprites.length-1
@character_sprites[index].dispose
@character_sprites.pop
end
end
#======================================
# ■ Scene_Map
#======================================
class Scene_Map
#--------------------------------------------------------------------------
alias abs_scene_map_update update
alias abs_scene_map_transfer_player transfer_player
#--------------------------------------------------------------------------
attr_accessor :spriteset
#--------------------------------------------------------------------------
def main
@spriteset = Spriteset_Map.new
@message_window = Window_Message.new
Graphics.transition
loop do
Graphics.update
Input.update
update
if $scene != self
break
end
end
Graphics.freeze
@spriteset.dispose
@message_window.dispose
if $scene.is_a?(Scene_Title)
Graphics.transition
Graphics.freeze
end
end
#--------------------------------------------------------------------------
def update
if Input.trigger?(Input::L)
$game_party.shift("Left")
end
if Input.trigger?(Input::R)
$game_party.shift("Right")
end
if Input.trigger?(Input::X)
$game_player.wait_command = true
$game_party.shift_forward
end
if Input.trigger?(Input::Y)
for ally in $game_allies.values
if ally.map_id == $game_map.map_id
ally.wait_command = false
end
end
end
for key in $game_allies.keys
$game_allies[key].update
end
abs_scene_map_update
end
#--------------------------------------------------------------------------
def transfer_player
for ally in $game_allies.values
if ally.wait_command == false
ally.moveto($game_temp.player_new_x, $game_temp.player_new_y)
ally.map_id = $game_temp.player_new_map_id
end
end
$game_player.map_id = $game_temp.player_new_map_id
abs_scene_map_transfer_player
end
end
#======================================
# ■ Scene_Title
#======================================
class Scene_Title
#--------------------------------------------------------------------------
alias abs_scene_title_cng command_new_game
#--------------------------------------------------------------------------
def command_new_game
$game_system.se_play($data_system.decision_se)
Audio.bgm_stop
Graphics.frame_count = 0
$game_temp = Game_Temp.new
$game_system = Game_System.new
$game_switches = Game_Switches.new
$game_variables = Game_Variables.new
$game_self_switches = Game_SelfSwitches.new
$game_screen = Game_Screen.new
$game_actors = Game_Actors.new
$game_party = Game_Party.new
$game_troop = Game_Troop.new
$game_map = Game_Map.new
$game_player = Game_Player.new
$game_allies = {}
staring_party_number = 0
for i in $data_system.party_members
staring_party_number += 1
$game_allies[staring_party_number] = Game_Ally.new(staring_party_number)
end
$game_party.setup_starting_members
$game_map.setup($data_system.start_map_id)
for ally in $game_allies.values
ally.moveto($data_system.start_x, $data_system.start_y)
ally.refresh
ally.map_id = $data_system.start_map_id
end
$game_player.map_id = $data_system.start_map_id
$game_player.moveto($data_system.start_x, $data_system.start_y)
$game_player.refresh
$game_map.autoplay
$game_map.update
$scene = Scene_Map.new
end
end
#======================================
# ■ Scene_Load
#======================================
class Scene_Load < Scene_File
#--------------------------------------------------------------------------
alias abs_scene_load_read_save_data read_save_data
#--------------------------------------------------------------------------
def read_save_data(file)
abs_scene_load_read_save_data(file)
$game_allies = Marshal.load(file)
if $game_system.magic_number != $data_system.magic_number
$game_map.setup($game_map.map_id)
$game_player.center($game_player.x, $game_player.y)
end
$game_party.refresh
end
end
#======================================
# ■ Scene_Save
#======================================
class Scene_Save < Scene_File
#--------------------------------------------------------------------------
alias abs_scene_save_write_save_data write_save_data
#--------------------------------------------------------------------------
def write_save_data(file)
abs_scene_save_write_save_data(file)
Marshal.dump($game_allies, file)
end
end